Problem: Right view of a binary tree is set of nodes visible when tree is visited from right side. Write a program to print the right view of a binary tree.
Example:
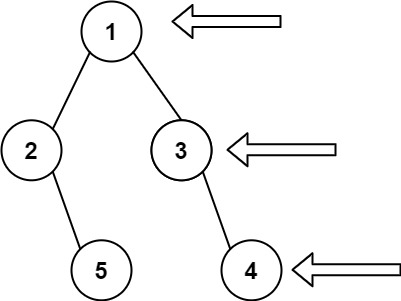
Input: root = [1,2,3,null,5,null,4] Output: [1,3,4]
Input: root = [1,null,3] Output: [1,3]
Input: root = [] Output: []
Implementation in C#:
public IList<int> RightSideView(TreeNode root)
{
List<int> result = new List<int>();
if (root == null)
{
return result;
}
Queue<TreeNode> queue = new Queue<TreeNode>();
queue.Enqueue(root);
while (queue.Count != 0)
{
int size = queue.Count;
TreeNode rightNode = null;
for (int i = 0; i < size; ++i)
{
rightNode = queue.Dequeue();
if (rightNode.left != null)
{
queue.Enqueue(rightNode.left);
}
if (rightNode.right != null)
{
queue.Enqueue(rightNode.right);
}
}
result.Add(rightNode.val);
}
return result;
}
No comments:
Post a Comment