Example:
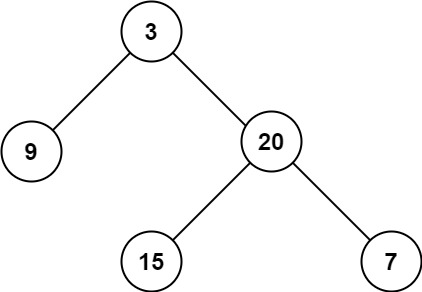
Input: root = [3,9,20,null,null,15,7] Output: 3
Input: root = [1,null,2] Output: 2
Approach: We can use post order traversal here. The simple formula of depth is Max(Depth(left), Depth(right) + 1. Obviously, if root itself is null then the depth is 0.
Implementation in C#:
public int MaxDepth(TreeNode root)
{
if (root == null)
{
return 0;
}
return Math.Max(this.MaxDepth(root.left),
this.MaxDepth(root.right)) + 1;
}
Complexity: O(n)